How to Initialize Multiple Variables in Java
- Java Howtos
- How to Initialize Multiple Variables in …

Importance of Initializing Multiple Variables with the Same Value
Initialize multiple string variables with the same value in java.
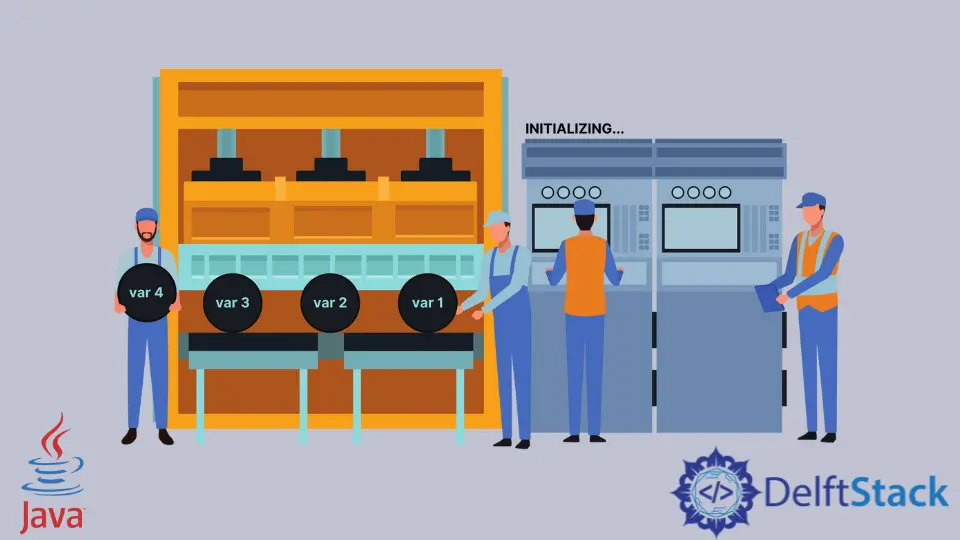
This article delves into the significance of initializing multiple variables with the same value and explores various methods to achieve this essential programming task.
Initializing multiple variables with the same value is essential for maintaining consistency and ensuring uniformity in a program. It simplifies code maintenance, reduces errors, and enhances readability.
When related variables share a common initial value, it fosters clarity in understanding code logic and promotes efficient use of resources. This practice is particularly crucial in scenarios where a group of variables should start with identical values, ensuring cohesive and predictable behavior in the program.
Inline Declaration and Initialization
The inline declaration and initialization method is crucial for concise code. Combining variable declaration and assignment in a single line minimizes redundancy and enhances readability.
This approach is efficient and effective, reducing the need for multiple lines and making it advantageous over alternative methods for initializing multiple variables with the same value.
Let’s consider a scenario where we need to initialize three integer variables ( a , b , and c ) with the same value (let’s say 10 ). Instead of repetitive individual assignments, we can leverage inline declaration and initialization to achieve this concisely.
In this code breakdown, we examine the significance of the inline declaration and initialization method.
Initially, three integer variables ( a , b , and c ) are declared and initialized in a single line, merging the variable type ( int ) with the assignment. This streamlined approach reduces code verbosity.
Subsequently, we display the initialized values of a , b , and c on the console, ensuring the successful implementation of the inline declaration and initialization method. This method proves crucial for maintaining code conciseness and verifying accurate variable initialization.
By employing inline declaration and initialization, we achieve a concise and expressive way of initializing multiple variables with the same value. The output of the provided code will be as follows:
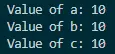
This output confirms that all three variables ( a , b , and c ) have been initialized with the desired value ( 10 ). Utilizing inline declaration and initialization not only enhances code readability but also promotes a more efficient and elegant coding style, making your Java programs more maintainable and less prone to errors.
Chained Assignments Method
The chained assignments method is pivotal for concise and readable code. It reduces redundancy by assigning values in a sequential manner, enhancing the efficiency of variable initialization.
This approach streamlines the process, making the code more compact and maintaining a clear logical flow, providing a distinct advantage over alternative methods.
Consider a scenario where three integer variables ( a , b , and c ) need to be initialized with the same value, let’s say 15 . The chained assignments method provides a concise and expressive way to achieve this:
Let’s analyze the code to comprehend the intricacies of the chained assignments method.
In the initial step, we declare and assign values to three integer variables ( a , b , and c ) in a single line, employing chained assignments. The process flows from right to left, with the rightmost value ( 15 ) assigned to c , followed by the value of c assigned to b , and ultimately, the value of b assigned to a .
Subsequently, we display the initialized values of a , b , and c on the console, ensuring the successful implementation of the chained assignments method for uniform initialization.
By employing the chained assignments method, we achieve a concise and expressive way of initializing multiple variables with the same value. The output of the provided code will be as follows:
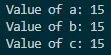
This output confirms that all three variables ( a , b , and c ) have been initialized with the desired value ( 15 ). The chained assignments method offers an elegant solution to streamline code while maintaining clarity, making it a valuable technique for enhancing the readability and efficiency of your Java programs.
Common Value Method
Initializing multiple variables with the same value using the common value method reduces redundancy and promotes code clarity by providing a single point of reference for initialization. This approach simplifies maintenance and ensures consistency, making code more concise and readable compared to other methods.
Consider a scenario where three integer variables ( a , b , and c ) need to be initialized with the same value, say 30 . The common value method provides a clean and concise solution:
In the code example, we employ the common value method to initialize multiple variables with the same value.
Initially, a variable named commonValue is declared and assigned the desired value ( 30 ). This single point of reference is then utilized to initialize three integer variables ( a , b , and c ).
By doing so, we minimize redundancy and enhance code clarity. The initialized values of these variables are subsequently displayed on the console.
This approach, known as the common value method, proves to be a simple and readable technique for achieving uniform initialization in Java.
By employing the common value method, we achieve a simple and effective way of initializing multiple variables with the same value. The output of the provided code will be as follows:
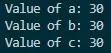
This output confirms that all three variables ( a , b , and c ) have been successfully initialized with the desired value ( 30 ). The common value method is particularly beneficial when dealing with a small number of variables, offering a clear and concise solution to the task of variable initialization in Java.
Array Method
The array method for initializing multiple variables with the same value is crucial for structured organization and uniformity. It minimizes redundancy, streamlines code, and provides a clear container for related variables.
This approach simplifies maintenance and promotes a concise, readable code structure, making it advantageous over other methods.
Imagine a scenario where you need to initialize three integer variables ( a , b , and c ) with the same value, say 20 . The array method provides an organized and efficient solution:
In the code, the array method for initializing multiple variables involves several essential steps.
Initially, an array of integers named variables is declared, providing a container for the related variables with a length of 3 . Following this, a variable named commonValue is declared and set to the desired shared value.
The core of the method lies in a for loop, where each element of the variables array is iterated, and the commonValue is assigned, achieving consistent initialization.
The code concludes by displaying the initialized values of the variables ( a , b , and c ). This method offers a structured approach, enhancing code organization and maintaining uniformity.
By employing the array method, we achieve an organized and efficient way of initializing multiple variables with the same value. The output of the provided code will be as follows:
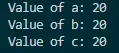
This output confirms that all three variables ( a , b , and c ) have been successfully initialized with the desired value ( 20 ). The array method provides a structured approach to handling related variables, contributing to cleaner and more maintainable Java code.
In conclusion, understanding the significance of initializing multiple variables with the same value is fundamental for code consistency and readability. This article has explored various effective methods, including inline declaration, chained assignments, common value, and array usage.
Choosing the right approach depends on the specific context of the program. By embracing these techniques, developers can streamline their code, reduce redundancy, and ensure a cohesive and predictable initialization of variables, contributing to the overall clarity and maintainability of Java programs.
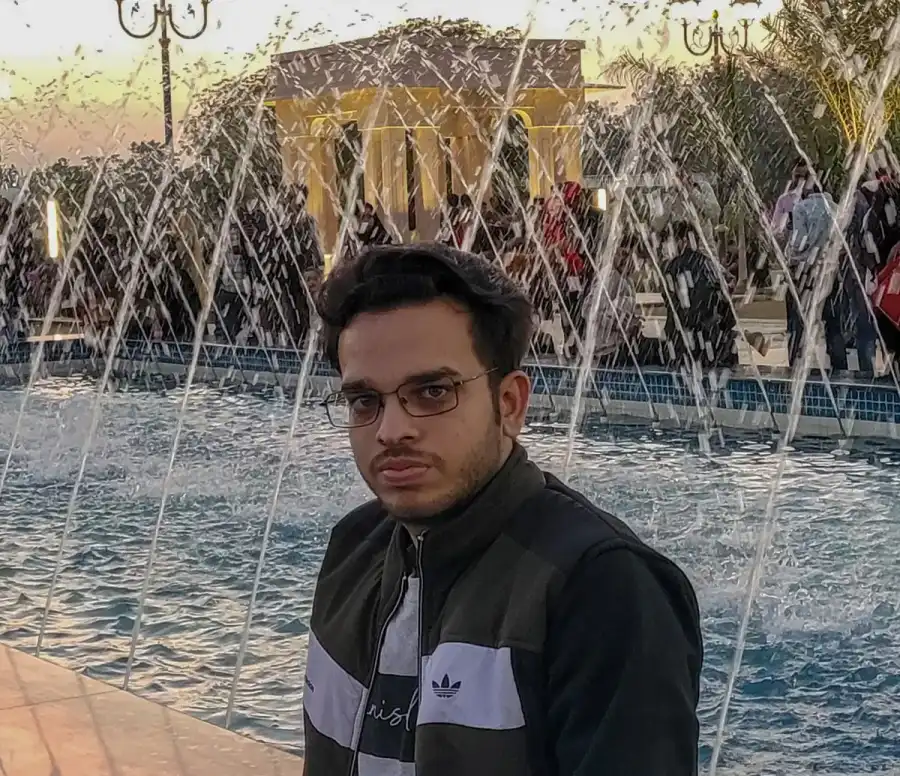
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
Related Article - Java Variable
- Java Synchronised Variable
- Difference Between Static and Final Variables in Java
- How to Set JAVA_HOME Variable in Java
- How to Create Counters in Java
- How to Increase an Array Size in Java
- How to Access a Variable From Another Class in Java
- Java Language Details
- For-Each Loop
- Loops and the Do-While Loop
- Switch Statement
- Jumping around - break, continue, return
Java Assignment Shortcuts
- More on Data Types
- Type Conversion and Casting
There are shortcuts in Java that let you type a little less code without introducing any new control structures.
Declaring and Assigning Variables You can declare multiple variables of the same type in one line of code:
You can also assign multiple variables to one value:
This code will set c to 5 and then set b to the value of c and finally a to the value of b .
Changing a variable One of the most common operations in Java is to assign a new value to a variable based on its current value. For example:
Since this is so common, Java let's you shorten it with a combined += operator that lets you skip the variable repetition:
(Note: Do not confuse this with index =+ 1; which would just assign positive 1 to index .)
In fact, any of the arithmetic operators can be used in this way:
j started at 3 and ended up at 5 after the above operations.
End of Free Content Preview. Please Sign in or Sign up to buy premium content.

- Trending Categories

- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Initializing multiple variables to the same value in Java
In this article, we will learn to initialize multiple variables to the same value in Java .
What is a variable?
A variable is a name given to a space reserved in the memory. Every variable has a type which specifies what kind of data it holds.

Initializing multiple variables to the same value
Multiple variables can be initialized to the same value in one statement as:
This means that the value is assigned to variable3, then variable3 is assigned to variable2, and finally, variable2 is assigned to variable1.
Java has two categories of data types primitive and non primitive. We will discuss the two categories separately.
Initialize multiple variables of primitive type at same time
Following are the steps to initialize multiple variables of primitive type at same time
- First, we will intialize the main class and then we will declare three integer variables a, b, and c are declared at the same time.
- Initialize the variables by assigning all three variables the same value (a = b = c = 10) .
- The value 10 is first assigned to c, then b gets the value of c, and finally, a gets the value of b.
- To display the values of a , b , and c we will print the values of it.
Java has eight types of primitive data types. Below is an example of assigning multiple primitive datatypes to the same value.
Since the assignment operator is right associative, firstly c is assigned the value of 1, then b is assigned the value of c and finally, a is assigned the value of b. In this case, three memory locations are created and the value 10 is copied to all three different memory locations.
Initialize multiple variables of non primitive type at same time
Following are the steps to initialize multiple variables of non primitive type at same time
- First, we will intialize the main class and then we will declare the three String variables str1 , str2 , and str3 are declared in the same statement.
- All three variables are assigned the same value (str1 = str2 = str3 = "Hello Java") .
- In this case, the memory for the string " Hello Java " is created once, and all three references (str1, str2, str3) point to the same location in memory.
- To print the values of the string variables we will print the values of it.
Non primitive data types like Strings, Arrays, and Classes can be assigned the same way as primitive datatypes. Below is an example of Strings:
The difference here is that the memory is allocated only once and the text "Hello Java" is copied to it.
Three references are created and all the references point to the same memory location.

- Related Articles
- Assign multiple variables to the same value in JavaScript?
- How to assign same value to multiple variables in single statement in C#?
- How to check multiple variables against a value in Python?
- Print Single and Multiple variables in Java
- Accessing variables of Int and Float without initializing in C
- Multiple Variables Holding Data - Which has the Highest Value in JavaScript?
- How to capture multiple matches in the same line in Java regex
- How to concatenate multiple string variables in JavaScript?
- Which MongoDB query finds same value multiple times in an array?
- MySQL query to find same value on multiple fields with LIKE operator?
- How to add a number to a current value in MySQL (multiple times at the same time)?
- Can we define multiple methods in a class with the same name in Java?
- Multiple index variables in PHP foreach loop
- Initializing HashSet in C#
- What kind of MongoDB query finds same value multiple times in an array?
Kickstart Your Career
Get certified by completing the course
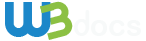
- Password Generator
- HTML Editor
- HTML Encoder
- JSON Beautifier
- CSS Beautifier
- Markdown Convertor
- Find the Closest Tailwind CSS Color
- Phrase encrypt / decrypt
- Browser Feature Detection
- Number convertor
- CSS Maker text shadow
- CSS Maker Text Rotation
- CSS Maker Out Line
- CSS Maker RGB Shadow
- CSS Maker Transform
- CSS Maker Font Face
- Color Picker
- Colors CMYK
- Color mixer
- Color Converter
- Color Contrast Analyzer
- Color Gradient
- String Length Calculator
- MD5 Hash Generator
- Sha256 Hash Generator
- String Reverse
- URL Encoder
- URL Decoder
- Base 64 Encoder
- Base 64 Decoder
- Extra Spaces Remover
- String to Lowercase
- String to Uppercase
- Word Count Calculator
- Empty Lines Remover
- HTML Tags Remover
- Binary to Hex
- Hex to Binary
- Rot13 Transform on a String
- String to Binary
- Duplicate Lines Remover
Initializing multiple variables to the same value in Java
In Java, you can initialize multiple variables to the same value in a single statement by separating the variables with a comma. Here is an example of how you can do this:
This code initializes three int variables, x , y , and z , to the value 10.
You can also use this syntax to initialize variables of different data types to the same value. For example:
This code initializes an int variable, x , to the value 10, a double variable, y , to the value 10.0, and a long variable, z , to the value 10L.
Note that you can only use this syntax to initialize variables to the same value. If you want to initialize variables to different values, you will need to use separate statements for each variable.
Related Resources
- Is Java "pass-by-reference" or "pass-by-value"?
- What are the differences between a HashMap and a Hashtable in Java?
- What is the difference between public, protected, package-private and private in Java?
- How do I determine whether an array contains a particular value in Java?
- What's the simplest way to print a Java array?
- How to get an enum value from a string value in Java
- A Java collection of value pairs? (tuples?)
- HTML Basics
- Javascript Basics
- TypeScript Basics
- React Basics
- Angular Basics
- Sass Basics
- Vue.js Basics
- Python Basics
- Java Basics
- NodeJS Basics

Declaring Multiple Variables in Java
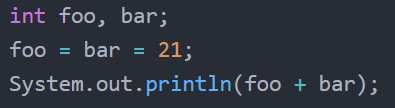
In Java , we can use a comma-separated list to simultaneously declare multiple variables of the same type. This can make it easy to declare sets of variables, reduces the size of our code and simplifies it.
Declaring Multiple Values Without Initializing
We can declare multiple variables of the same type on a single line without initializing them . The following example demonstrates this:
In this example, we have declared two integer variables foo and bar . We’ve left them uninitialized for now, but we will need to do so at some point before attempting to call them.
Declaring and Initializing Multiple Variables
We can also declare and initialize variables of the same type on a single line using the assignment ‘=’ operator:
Output : 42
In this case, we’ve successfully initialized foo to a value of 12 and bar to a value of 30. We then printed the sum of foo and bar to the console using System.out.println() .
Declare and Initialize Multiple Variables to the Same Value
If we have multiple variables of the same type that need to be initialized to the same value, we can declare them all on one line and then initialize them all on a separate line:
The output of this program is 57 , because the first time we print fred his value is 5, and the second time his value is 7.
This kind of multiple assignment is the reason I described variables as a container for values. When you assign a value to a variable, you change the contents of the container, as shown in the figure:
When there are multiple assignments to a variable, it is especially important to distinguish between an assignment statement and a statement of equality. Because Java uses the = symbol for assignment, it is tempting to interpret a statement like a = b as a statement of equality. It is not!
First of all, equality is commutative, and assignment is not. For example, in mathematics if a = 7 then 7 = a . But in Java a = 7; is a legal assignment statement, and 7 = a; is not.
Furthermore, in mathematics, a statement of equality is true for all time. If a = b now, then a will always equal b . In Java, an assignment statement can make two variables equal, but they don't have to stay that way!
The third line changes the value of a but it does not change the value of b , and so they are no longer equal. In many programming languages an alternate symbol is used for assignment, such as <- or := , in order to avoid this confusion.
Although multiple assignment is frequently useful, you should use it with caution. If the values of variables are changing constantly in different parts of the program, it can make the code difficult to read and debug.

IMAGES
COMMENTS
Jun 1, 2016 · You can declare multiple variables, and initialize multiple variables, but not both at the same time: String one,two,three; one = two = three = ""; However, this kind of thing (especially the multiple assignments) would be frowned upon by most Java developers, who would consider it the opposite of "visually simple".
Feb 14, 2024 · Initialize Multiple String Variables With the Same Value in Java Inline Declaration and Initialization. The inline declaration and initialization method is crucial for concise code. Combining variable declaration and assignment in a single line minimizes redundancy and enhances readability.
You can also assign multiple variables to one value: a = b = c = 5; This code will set c to 5 and then set b to the value of c and finally a to the value of b. Changing a variable One of the most common operations in Java is to assign a new value to a variable based on its current value. For example: int index = 0; index = index + 1;
Sep 23, 2024 · Initializing multiple variables to the same value in Java - In this article, we will learn to initialize multiple variables to the same value in Java. What is a variable? A variable is a name given to a space reserved in the memory. Every variable has a type which specifies what kind of data it holds. Initializing multiple variables to the same value Multipl
This code initializes an int variable, x, to the value 10, a double variable, y, to the value 10.0, and a long variable, z, to the value 10L. Note that you can only use this syntax to initialize variables to the same value. If you want to initialize variables to different values, you will need to use separate statements for each variable.
Declaring Multiple Variables in Java Fig 1: Declaring two variables on one line and initializing them together on a second line. In Java, we can use a comma-separated list to simultaneously declare multiple variables of the same type. This can make it easy to declare sets of variables, reduces the size of our code and simplifies it.
Apr 14, 2023 · One Value to Multiple Variables. In Java, you can assign one value to multiple variables at once. Here are the steps to do this: Step 1: Choose the data type. Choose the data type of the variables you want to assign the value to. Step 2: Declare the variables. Declare the variables by listing their names, separated by commas.
When there are multiple assignments to a variable, it is especially important to distinguish between an assignment statement and a statement of equality. Because Java uses the = symbol for assignment, it is tempting to interpret a statement like a = b as a statement of equality. It is not! First of all, equality is commutative, and assignment ...
Sep 29, 2024 · Java doesn’t support assigning multiple values to variables on a single line like Python. This article explains why, offers correct ways to declare variables in Java and also highlights best practices to enhance readability and avoid variable errors. Follow these guidelines to write cleaner, more maintainable Java code.
May 15, 2015 · You can define multiple variables like this : double a,b,c; Each variable in one line can also be assigned to specific value too: double a=3, b=5.2, c=3.5/3.5; One more aspect is, while you are preparing common type variable in same line then from right assigned variables you can assign variable on left, for instance : int a = 4, b = a+1, c=b*b;